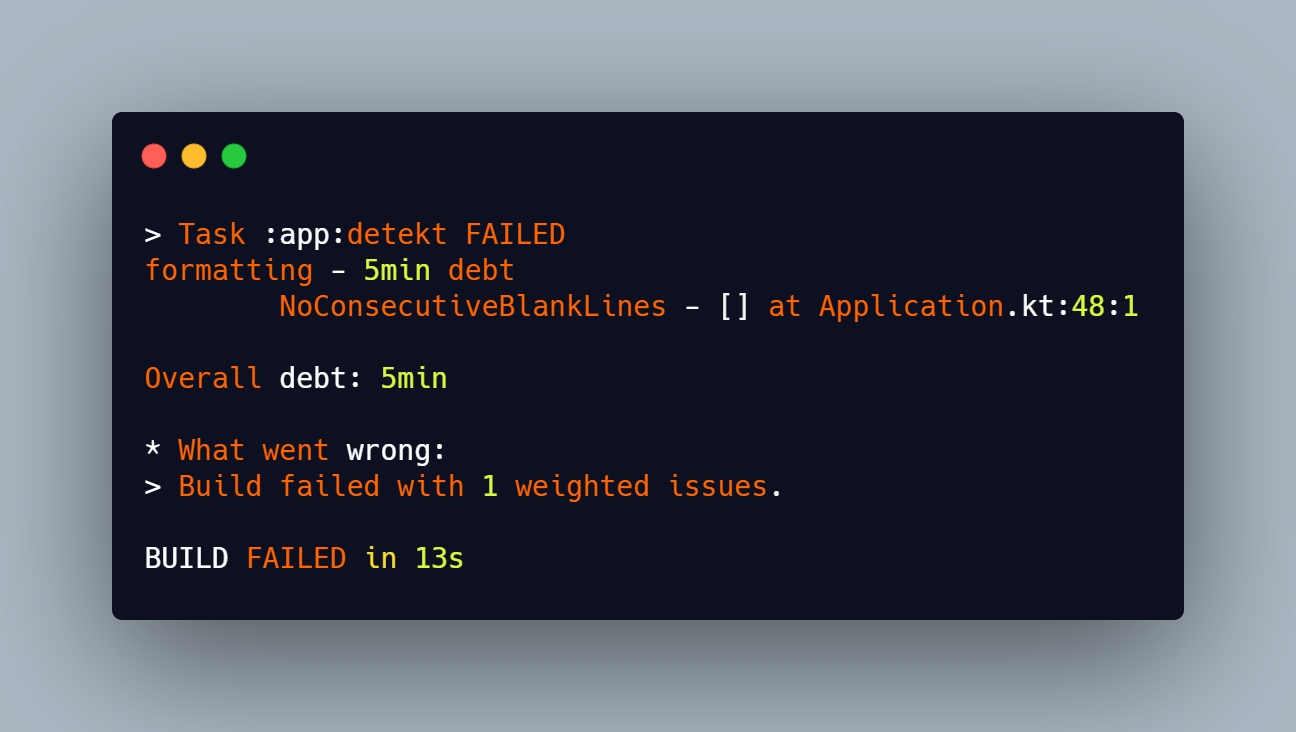
Overview
There are a number of things that could slow down an Android dev team. It could be the time wasted troubleshooting a buggy codebase, navigating through an ill-structured set of classes and having to do a lot of refactoring to add new features. All of these correlate to how well the product is implemented and the amount of technical debt it has accumulated throughout its time. Hence making software quality of the essential attributes to the success of a product.
When developing software products, teams often require feedback prior to merging code. This ensures the changes adhere to the best standards and follow specifications that encourage maintainability and flexibility of new requirements. The manual review process is an important act of collaboration which is an entry point to catching a lot of the technical issues that could impact users. Teams often use static code analysis tools that scan the codebase and highlight issues and warnings of bad implementation practices within the codebase. These automated checks are fast and reliable, hence reducing the feedback cycle and allowing better code to be merged into the code repository.
Linting on Android is no different than any other development framework. It can help you and your team with:
- Standardizing a coding design structure
- Highlighting coding errors and bad practices
- Calculating cyclomatic complexity
- Prompting warnings of deprecated dependencies and unused resources
- Pinpointing accessibility and localization issues (and much more...)
Linting - It's a second pair of eyes that provides additional insights on what can be improved in the codebase adhering to a high quality of software development.
Detekt
Detekt is a configurable lint tool for Kotlin that can be used within your Android project. It comes with a predefined set of rules that analyzes potential code smells. Upon integrating detekt, a Gradle task is created that can run the analysis and output a report containing the list of current issues. This task can be executed locally and on a continuous integration (CI) pipeline.
To integrate detekt in your project, make the following changes:
/** build.gradle (project) */
plugins {
id "io.gitlab.arturbosch.detekt" version "1.18.1"
}
/** build.gradle (app) */
apply plugin: 'io.gitlab.arturbosch.detekt'
dependencies {
// optional: additional formatting rules from ktlint
detektPlugins("io.gitlab.arturbosch.detekt:detekt-formatting:1.18.1")
}
// detekt configuration block.
detekt {
toolVersion = "1.18.1"
basePath = projectDir
reports {
html { enabled = true }
txt { enabled = false }
xml { enabled = false }
}
}
To run the detekt lint analysis, open the terminal and navigate to the project directory. Then execute ./gradlew detekt
Lint Issues
After running detekt, you will see a lot of lint issues in the report. This is normal! By design, detekt is using the default ruleset which can be customized as well as allowing some rules to be disabled. Ideally, you should not try to fix all of the lint errors at once. Instead, analyze the lint report and determine which issues are important that need to be fixed and which can be turned off and ignored. With detekt, you can configure the threshold, disable a rule, suppress the issue within the codebase or add that issue to the baseline. More on this later.
Here is an example of what you can expect from your lint report.
These reports are generated in the app/build/reports folder.
Advantages
A tool like detekt can recommend a lot of great suggestions in improving the quality of the codebase. Here are a few advantages of using detekt in your development workflow:
- Automatically find issues that could turn into potential bugs
- Generates an useful report that provides additional insights
- Allow team members to follow a standard when contributing to the codebase
- Highly configurable ruleset that can be adjusted and turned off
- Ability to fail the CI pipeline if an issue is found
That's a wrap! Stay tuned for more blog posts around detekt.